Cryptoticker
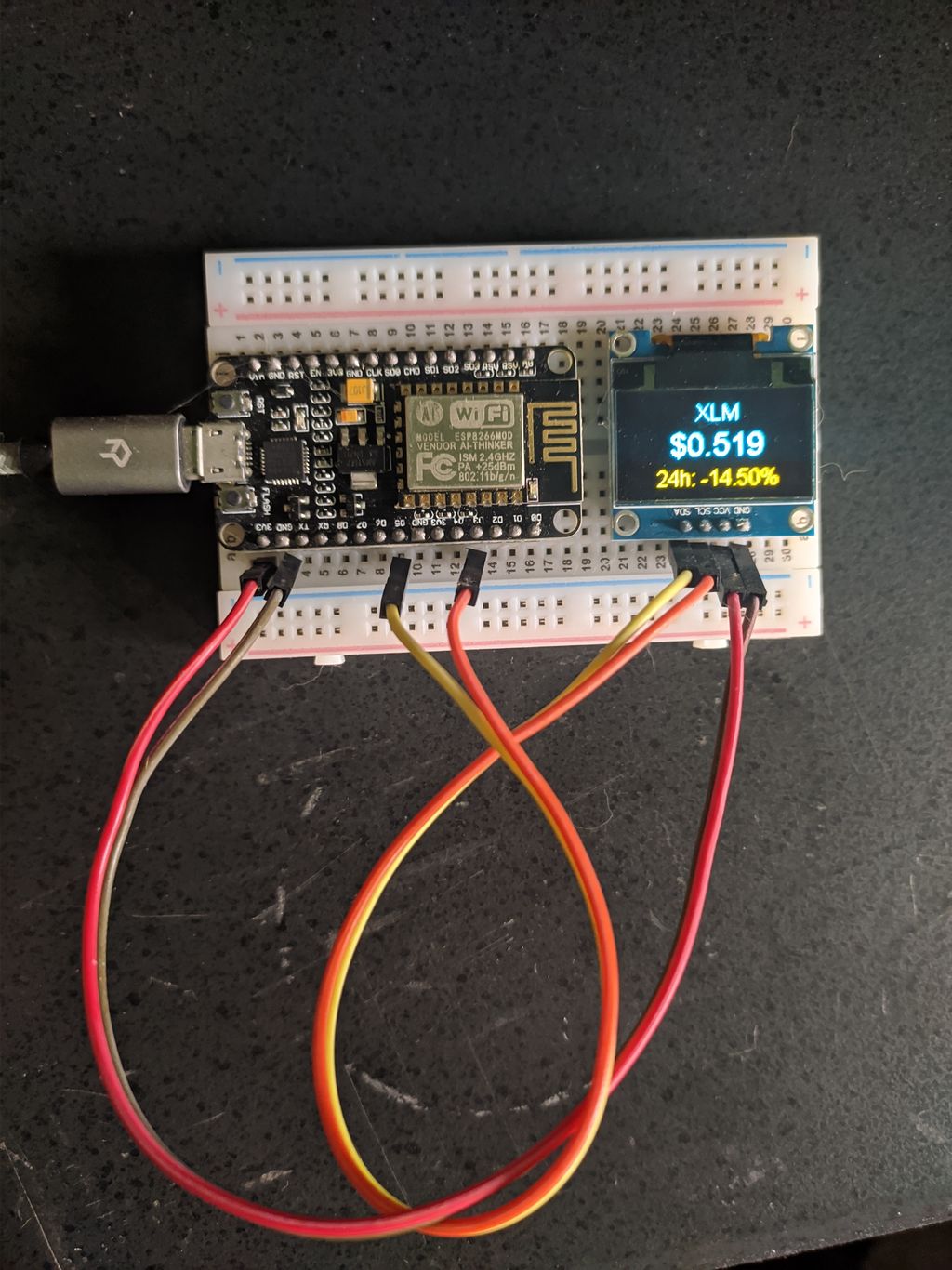
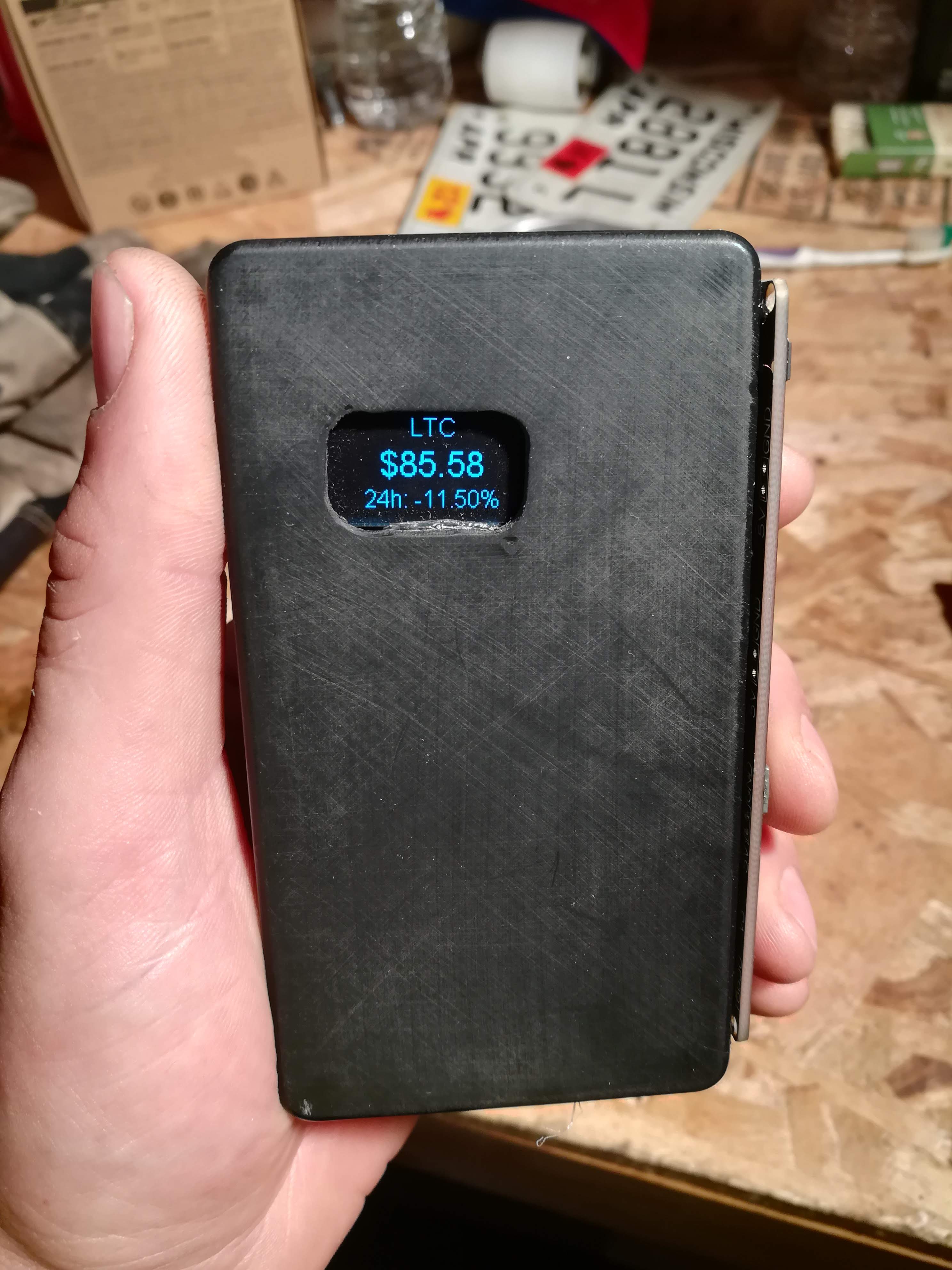
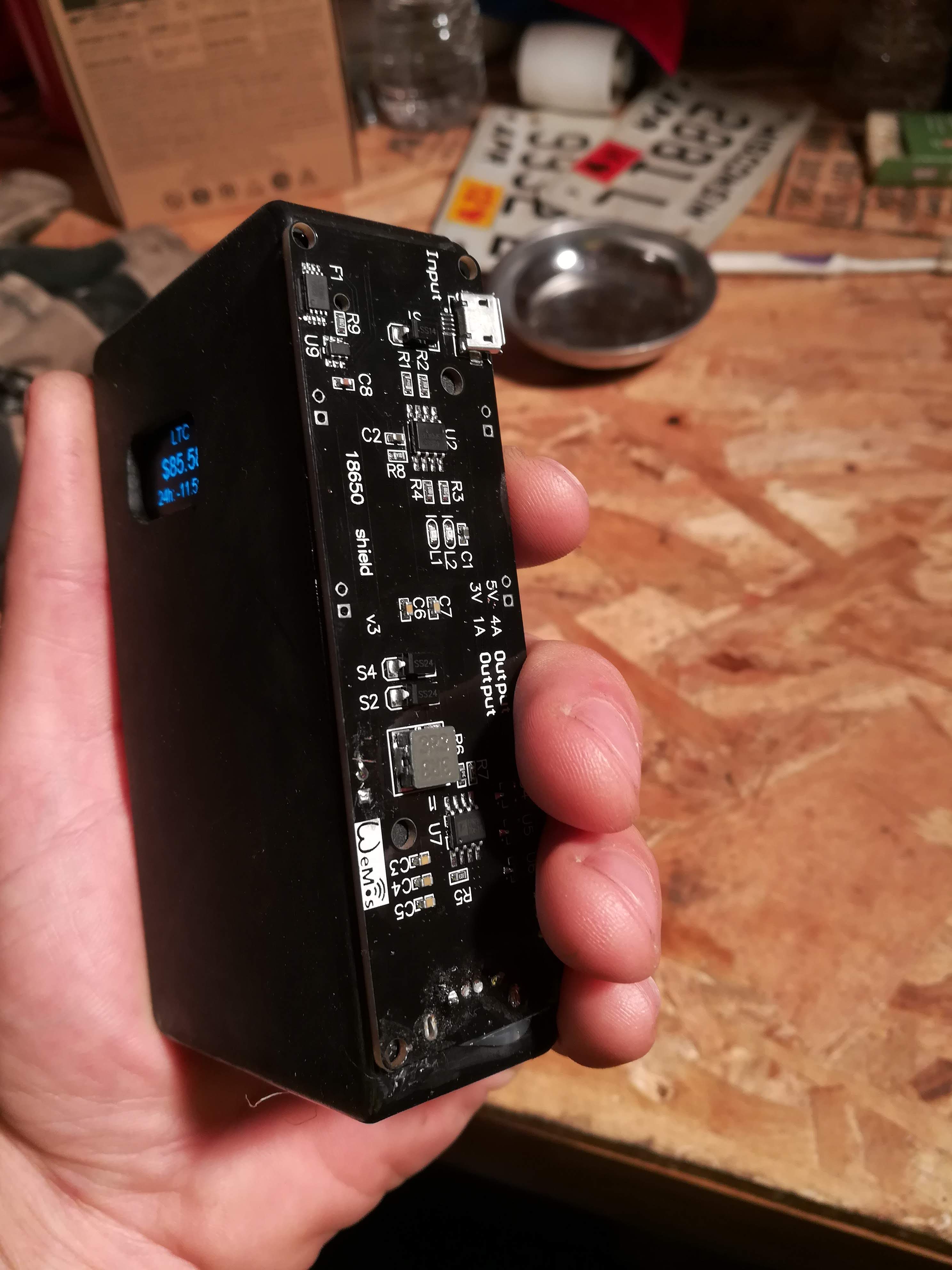
I tried making some minor changes and updating my cryptoticker and it failed with, “error loading data.”
After some searches I found this open ticket on github, https://github.com/witnessmenow/arduino-coinmarketcap-api/issues/4
Although it was working before hand, it should still have worked and I was stumped as to why it was failing. Some comments mentioned the API had changed, another comment mentioned it was still working for them. I decided to rewrite the code using a different, free API, as suggested per this comment: https://github.com/witnessmenow/arduino-coinmarketcap-api/issues/4#issuecomment-444731941
Here is what I came up with:
#include <ESP8266WiFi.h>
#include <ESP8266HTTPClient.h>
#include <ArduinoJson.h> //need to use version < 6, I'm using version 5.12.0
#include "SH1106Wire.h"
#include <DNSServer.h>
#include <ESP8266WebServer.h>
#include <WiFiManager.h> //https://github.com/tzapu/WiFiManager
// Pins based on your wiring
#define SCL_PIN D5
#define SDA_PIN D3
//setup display
SH1106Wire display(0x3c, SDA_PIN, SCL_PIN);
//global variables
float eth_usd;
float eth_usd_24h_change;
void setup() {
Serial.begin(115200);
//start the WiFiManager
WiFiManager wifiManager;
wifiManager.autoConnect("CryptoTicker"); //if no known WiFi connections available, creates CryptoTicker WiFi which can be used to set the cryptoticker to connect to another WiFi from your phone, for me it set to 192.168.4.1
while (WiFi.status() != WL_CONNECTED) {
Serial.println("WiFi Connected.");
}
//boot title
display.init();
display.setTextAlignment(TEXT_ALIGN_CENTER);
display.setFont(ArialMT_Plain_16);
display.drawString(64, 20, F("CryptoTicker"));
display.display();
delay(2000);
}
//main
void loop() {
// Check WiFi Status
if (WiFi.status() == WL_CONNECTED) {
Serial.println("Connected!");
HTTPClient http;
//using the coingecko api, see their documentation, to add coins seperate with commas
//using https://www.coingecko.com/api/documentations/v3 -- they have a URL generator, but basically just seperate your currencies with commas, example: ripple,bitcoin,litecoin,ethereum in the URL
//I found the URL has to end in a trailing slash or it won't work and will return a -1 error code for the http response so I added &/ on the end of the URL
String api="https://api.coingecko.com/api/v3/simple/price?ids=ethereum&vs_currencies=usd&include_24hr_change=true&/";
//used https://www.grc.com/fingerprints.htm to get SSL fingerprint for the JSON URL used above
String thumbprint="C7:1C:BC:99:48:E9:56:E2:E7:4F:8D:EB:5B:39:3E:6E:27:1F:35:81";
http.begin(api, thumbprint);
//http.addHeader("Content-Type", "application/json");
int httpCode = http.GET();
Serial.println(httpCode);
//Check the returning code
if (httpCode == HTTP_CODE_OK) {
// Parsing
// generated with https://arduinojson.org/v5/assistant/
// regenerate with new api URL if adding coins - it will set a variable for each item, need to manually add global variables at the top
//from here -------------------------
const size_t capacity = JSON_OBJECT_SIZE(1) + JSON_OBJECT_SIZE(2) + 60;
DynamicJsonBuffer jsonBuffer(capacity);
String payload = http.getString();
JsonObject& root = jsonBuffer.parseObject(payload);
eth_usd = root["ethereum"]["usd"];
eth_usd_24h_change = root["ethereum"]["usd_24h_change"];
//to here -------------------------
}
http.end(); //Close connection
}
displayValue();
displayHolding();
}
//display value of coin and 24 hour change
//copa pasta code with new variables if adding coins
void displayValue(){
display.clear();
display.setTextAlignment(TEXT_ALIGN_CENTER);
display.setFont(ArialMT_Plain_16);
display.drawString(64, 0, F("ETH"));
display.setFont(ArialMT_Plain_24);
display.drawString(64, 20, "$" + String(eth_usd));
display.setFont(ArialMT_Plain_16);
display.drawString(64, 48, "24h: " + String(eth_usd_24h_change) + "%");
display.display();
delay(10000); //display current price for this amount of time
}
//display my holdings
void displayHolding(){
display.clear();
int holdings = 8.22; //set this to the amount of coins you have
int value = eth_usd*holdings;
display.setTextAlignment(TEXT_ALIGN_CENTER);
display.setFont(ArialMT_Plain_16);
display.drawString(64, 0, F("ETH"));
display.setFont(ArialMT_Plain_24);
display.drawString(64, 20, "$" + String(value));
display.display();
delay(3000); // display holdings for this amount of time
}